We
will learn how to automate Docker builds using Jenkins and Deploy into
AWS EKS - Kubernetes Cluster. We will use Python based container application. I have already
created a repo with source code + Dockerfile. The repo also have
Jenkinsfile for automating the following:
- Automating builds using Jenkins
- Automating Docker image creation
- Automating Docker image upload into Docker registry
- Automating Deployments to Kubernetes Cluster
1. Amazon EKS Cluster is setup and running. Click here to learn how to create Amazon EKS cluster.
4. Docker, Docker pipeline and Kubernetes Deploy plug-ins are installed in Jenkins
Step #1 -Make sure Jenkins can run Docker builds after validating per pre-requisites
Click on Global credentials
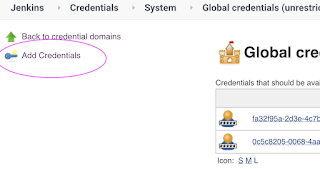
Now Create an entry for your Docker Hub account. Make sure you enter the ID as dockerhub
Step #4 - set a clusterrole as cluster-admin
By default, clusterrolebinding has system:anonymous set which blocks the cluster access. Execute the following command to set a clusterrole as cluster-admin which will give you the required access.
Make sure you change red highlighted values below:
Your docker user id should be updated.
your registry credentials ID from Jenkins from step # 1 should be copied
label 'myslave'
}
environment {
//once you sign up for Docker hub, use that user_id here
registry = "your_docker_hub_user_id/mypython-app"
//- update your credentials ID after creating credentials for connecting to Docker Hub
registryCredential = 'dockerhub'
dockerImage = ''
}
stages {
stage ('checkout') {
steps {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [], userRemoteConfigs: [[url: 'https://github.com/akannan1087/myPythonDockerRepo']]])
}
}
stage ('Build docker image') {
steps {
script {
dockerImage = docker.build registry
}
}
}
// Uploading Docker images into Docker Hub
stage('Upload Image') {
steps{
script {
docker.withRegistry( '', registryCredential ) {
dockerImage.push()
}
}
}
}
stage ('K8S Deploy') {
steps {
script {
kubernetesDeploy(
configs: 'k8s-deployment.yaml',
kubeconfigId: 'K8S',
enableConfigSubstitution: true
)
}
}
}
}
}
No comments:
Post a Comment