We
will learn how to automate Docker builds using Jenkins and Deploy into
Kubernetes Cluster. We will use Python based application. I have already
created a repo with source code + Dockerfile. The repo also have
Jenkinsfile for automating the following:
- Automating builds using Jenkins
- Automating Docker image creation
- Automating Docker image upload into Docker registry
- Automating Deployments to Kubernetes Cluster
Pre-requisites:
1. Jenkins Master is up and running.
1. Jenkins Master is up and running.
2. Spin up Jenkins slave, install docker in it. Install kubectl on Slave.
3. Docker, Docker pipeline and Kubernetes Deploy plug-ins are installed in Jenkins
3. Docker, Docker pipeline and Kubernetes Deploy plug-ins are installed in Jenkins
5. Kubernetes Cluster is setup and running.
Step #1 - Login to Jenkins slave instance through command line - Install Docker
Step #1 - Login to Jenkins slave instance through command line - Install Docker
sudo apt install docker.io -y
Add Jenkins to Docker Group
sudo usermod -aG docker jenkins
sudo systemctl daemon-reload
sudo usermod -aG docker jenkins
sudo systemctl daemon-reload
Restart Docker service
sudo systemctl start docker
sudo systemctl enable dockersudo systemctl restart docker
Restart Jenkins service
sudo service jenkins restart
Install kubectl command on Jenkins slave.
Step #2 - Create Credentials for Docker Hub
Go to Jenkins UI, click on Credentials -->
Click on Global credentials
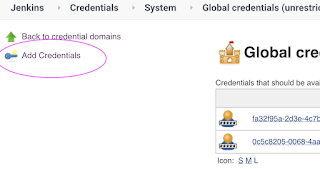
Now Create an entry for your Docker Hub account. Make sure you enter the ID as dockerhub
Step #3 - Create Credentials for Kubernetes Cluster
Click on Global credentials
Click on Add Credentials
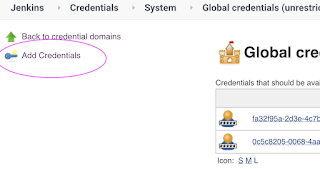
Now Create an entry for your Docker Hub account. Make sure you enter the ID as dockerhub
execute the below command to get kubeconfig info, copy the entire content of the file:
sudo cat ~/.kube/config
Go to Jenkins, Manage Jenkins, Click on Add Credentials, use Kubernetes configuration from drop down.
Enter ID as kubeconfig_raw and upload kubeconfig file
Upload kube config and enter id as ID kubeconfig-raw
Step # 4 - Create a pipeline in Jenkins
Step # 5 - Copy the pipeline code from below
Make sure you change red highlighted values below:
Your docker user id should be updated.
your registry credentials ID from Jenkins from step # 1 should be copied
label 'myslave'
}
environment {
//once you sign up for Docker hub, use that user_id here
registry = "your_docker_hub_user_id/mypython-app"
//- update your credentials ID after creating credentials for connecting to Docker Hub
registryCredential = 'dockerhub'
dockerImage = ''
}
stages {
stage ('checkout') {
steps {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [], userRemoteConfigs: [[url: 'https://github.com/akannan1087/myPythonDockerRepo']]])
}
}
stage ('Build docker image') {
steps {
script {
dockerImage = docker.build registry
}
}
}
// Uploading Docker images into Docker Hub
stage('Upload Image') {
steps{
script {
docker.withRegistry( '', registryCredential ) {
dockerImage.push()
}
}
}
}
stage('K8S Deploy') {
steps{
script {
withKubeConfig([credentialsId: 'kubeconfig-raw', serverUrl: '']) {
sh ('kubectl apply -f k8s-deployment.yaml')
}
}
}
}
}
}
Make sure you change red highlighted values below:
Your docker user id should be updated.
your registry credentials ID from Jenkins from step # 1 should be copied
pipeline {
agent {label 'myslave'
}
environment {
//once you sign up for Docker hub, use that user_id here
registry = "your_docker_hub_user_id/mypython-app"
//- update your credentials ID after creating credentials for connecting to Docker Hub
registryCredential = 'dockerhub'
dockerImage = ''
}
stages {
stage ('checkout') {
steps {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [], userRemoteConfigs: [[url: 'https://github.com/akannan1087/myPythonDockerRepo']]])
}
}
stage ('Build docker image') {
steps {
script {
dockerImage = docker.build registry
}
}
}
// Uploading Docker images into Docker Hub
stage('Upload Image') {
steps{
script {
docker.withRegistry( '', registryCredential ) {
dockerImage.push()
}
}
}
}
stage('K8S Deploy') {
steps{
script {
withKubeConfig([credentialsId: 'kubeconfig-raw', serverUrl: '']) {
sh ('kubectl apply -f k8s-deployment.yaml')
}
}
}
}
}
}
Step # 6 - Build the pipeline
Once you create the pipeline and changes values per your Docker user id and credentials ID, click on
Step # 7 - Verify deployments to K8S
kubectl get pods
kubectl get deployments
Steps # 8 - Access Python App in K8S cluster
Once build is successful, go to browser and enter master or worker node public ip address along with port number mentioned above
http://master_or_worker_node_public_ipaddress:port_no_from_above
You should see page like below:
No comments:
Post a Comment